[SpringBoot]整理Controller层遇到的注解
前提:
SpringBoot用起来是很方便,但是太多的注解有时候会搞混,自己用起来也是哪个搞不清了就google一下,所以干脆自己总结一下
一、注解Controller类
1.@Controller
@Controller public class HelloController { @RequestMapping("/hello") public String sayHello() { return "hello world"; } }
如果直接使用@Controller
在浏览器输入http://127.0.0.1:8082/hello
会出现报错
原因是因为@Controller
注解必须配合模板来使用,也就是@Controller
是来响应界面的,如果要使用可以使用Thymeleaf
模板(Spring推荐)。
在pom.xml
中加入以下依赖
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency>
在resources->templates目录下添加hello.html文件,随便编写内容再次运行就会正常。
注:Controller层中的【return ”hello“】hello就是页面的名称。
2.@RestController
@RestController public class HelloController { @RequestMapping("/hello") public String sayHello() { return "hello world"; } }
spring 4 新加注解,这个注解相当于@Controller+@ResponseBody,主要是使 http 请求返回数据格式为 json 格式,正常情况下都是使用这个注解。
二、URL注解
1.@RequestMapping
RequestMapping是一个用来处理请求地址映射的注解,可用于类或方法上。用于类上,表示类中的所有响应请求的方法都是以该地址作为父路径。
@RestController @RequestMapping("/test") public class HelloController { @RequestMapping("/hello") public String sayHello() { return "hello world"; } }
如上高亮的那个2行就是地址的映射注解,访问地址为
http://127.0.0.1:8082/test/hello
该注解有六个属性:
params:指定request中必须包含某些参数值是,才让该方法处理。
headers:指定request中必须包含某些指定的header值,才能让该方法处理请求。
value:指定请求的实际地址。
method:指定请求的method类型, GET、POST、PUT、DELETE等。
consumes:指定处理请求的提交内容类型(Content-Type),如application/json或者text/html。
produces:指定返回的内容类型,仅当request请求头中的(Accept)类型中包含该指定类型才返回。
举个例子
@RestController public class HelloController { @RequestMapping(params = "test=mTest,", value = "/hello", method = RequestMethod.GET, headers = "Referer=http://127.0.0.1", consumes = "application/json", produces = "application/json") public String sayHello() { return "hello world"; } }
一般常用的也就是value,method
2.地址映射的组合注解
@GetMapping
和@PostMapping
等由请求方法(get,post)+Mapping等价于@RequestMapping(method=RequestMethod.POST)
在举个例子
@RequestMapping(value="/hello" method=RequestMethod.POST)
等于
@PostMapping(value="/hello")
三、参数注解
1.@PathVariable
在Url中拼接一个字符串{name},用户在请求是添加
@RestController public class HelloController { @GetMapping("/hello/{name}") public String sayHello(@PathVariable("name") String name) { return "hello-" + name; } }
在浏览器访问http://127.0.0.1:8082/hello/leeyk
输出结果如下图
2.@RequestParam
获取请求参数值,一般都用post来提交,注意这里指的是body的值。
@RestController public class HelloController { @PostMapping("/hello") public String sayHello(String name) { return "hello-" + name; } }
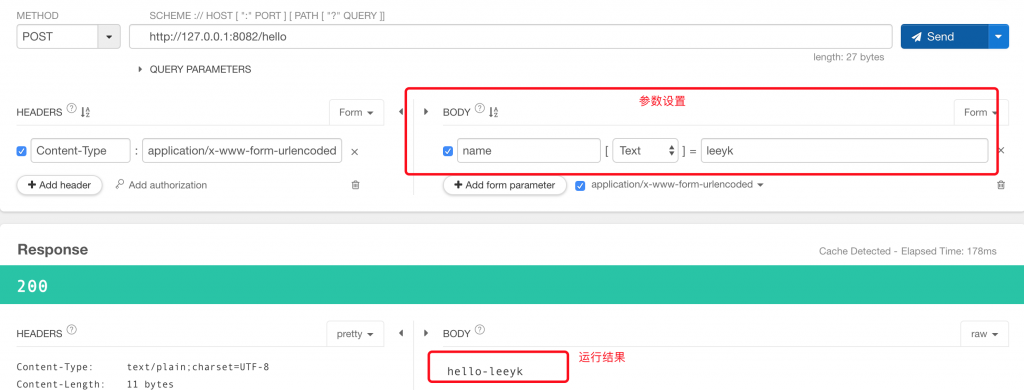
@RestController public class HelloController { @PostMapping("/hello") public String sayHello(@RequestParam("newName") String name) { return "hello-" + name; } }
意思就是表单的参数为newName,可我们后台要用name来接收,就要像上图一样写,运行结果如下
如果我们还用name来传值就会报错:
正确方法如下:
同理get提交也是一样,都支持写与不写@RequestParam,但是请求时就是要拼接url
代码如下:
@RestController public class HelloController { @GetMapping("/hello") public String sayHello(@RequestParam("newName") String name) { return "hello-" + name; } }
运行结果如下:
注:如果用户没有传参数,就会报404
错误。再添加两个设置 required = false
:是否必传;defaultValue
:默认值。
@RequestParam( required = false,defaultValue = "leeyk",value = "newName")
3.@RequestHeader
获取头部参数
@RestController public class HelloController { @GetMapping("/hello") public String sayHello(@RequestHeader String name) { return "hello-" + name; } }
4.@RequestBody
在Controller方法带有@RequestBody注解的参数,意味着请求的HTTP消息体的内容是一个JSON.
springboot默认使用Jackson来处理序列化和反序列化.
@RequestBody接收数据时,前端不能使用GET方式提交数据,而是用POST方式进行提交.
@RestController public class HelloController { @PostMapping("/hello") public String sayHello(@RequestBody User user) { return "hello-" + user.getName(); } }
请求数据可以是json
Tom
2019年11月20日 下午3:45
写的不错